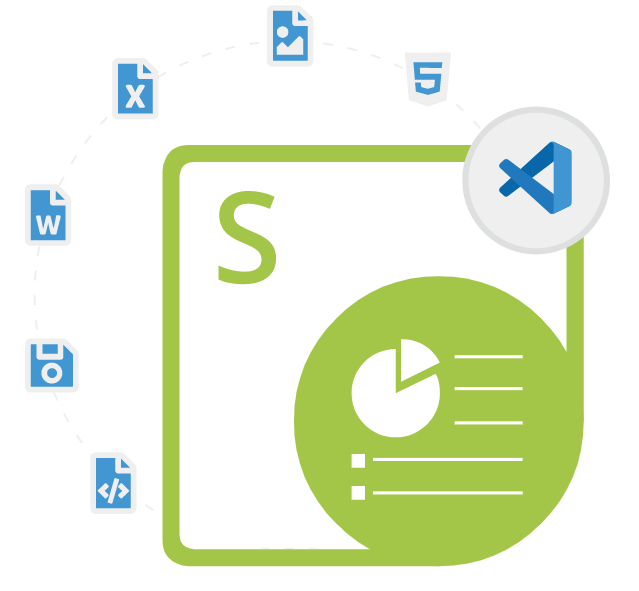
Aspose.Slides for .NET
C# .NET API to Create & Convert Excel Spreadsheets
Advanced PowerPoint presentation API to generate, edit, merge, protect, convert, & render PPT/PPTX files without using Microsoft PowerPoint.
Aspose.Slides for .NET is a very useful PowerPoint presentation creation and manipulation library without using Microsoft PowerPoint or any other external dependencies. The library allows developers to create interactive and engaging presentations that can be used for a variety of purposes, such as presentations, reports, and marketing materials. The library has included support for various PowerPoint presentations & OpenOffice file formats such as PPT, PPTX, PPS, POT, PPSX, PPTM, PPSM, POTX, POTM, and ODP.
Aspose.Slides for .NET has included a wide range of basic as well as advanced features for handling presentations such as creating presentations from scratch, adding new slides, cloning slides, copying slides, modifying a presentation’s document properties, deleting unwanted slides, copying masters, preview slides¸ export slides as images, export slides to SVG format, managing slide notes, setting new slide masters, add slide notes, creating or managing shapes, manage styles in shapes, creating tables from scratch, resizing and moving tables, changing the text formatting and many more.
Aspose.Slides for .NET has included a very powerful converter that helps software developers to export presentations into some leading file formats such as PDF, PDF/A, XPS, HTML, and popular images file formats like JPEG, PNG, BMP, TIFF, and GIF. It also provides a number of advanced capabilities, such as support for comments, slide transitions, and animation. The library has included support for a wide range of PowerPoint elements, including slides, shapes, text, images, charts, and tables. This allows developers to easily add, edit, and format these elements within their presentations.
Getting Started with Aspose.Slides for .NET
The recommend way to install Aspose.Slides for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Slides for .NET via NuGet
NuGet\Install-Package Aspose.Slides.NET -Version 23.1.0
You can also download it directly from Aspose product release page.Generate & Manage Presentations via .NET API
Aspose.Slides for .NET has included the capability to create and manipulate PowerPoint presentations inside .NET applications without any external dependencies. Developers can also open existing presentations and make changes to them with ease. It is also possible to check the format of a presentation and examine it to find out its properties and understand its behavior. It is also possible to get the properties of a presentation and update it.
Creating Presentations via .NET API
Presentation presentation = new Presentation();
presentation.Save("OutputPresenation.pptx", SaveFormat.Pptx);
Convert PowerPoint Presentations via .NET API
One of the key features of Aspose.Slides for .NET is the conversion of PowerPoint presentations to a wide variety of output formats, including PDF, HTML, PDF/A, XPS, SVF, GIF, TIFF, PNG, BMP, and JPEG quickly and easily. This makes it easy to convert presentations to a format that is compatible with a specific application or platform. Additionally, it also provides a number of advanced options for converting presentations, such as setting image quality and resolution, specifying page size and orientation, and adding watermarks to the output file. The library also provides the ability to convert a PowerPoint slide to an image.
Convert PowerPoint to PDF with Hidden Slides via .NET API
// Instantiates a Presentation class that represents a PowerPoint file
Presentation presentation = new Presentation("PowerPoint.pptx");
// Instantiates the PdfOptions class
PdfOptions pdfOptions = new PdfOptions();
// Adds hidden slides
pdfOptions.ShowHiddenSlides = true;
// Saves the presentation as a PDF
presentation.Save("PowerPoint-to-PDF.pdf", SaveFormat.Pdf, pdfOptions);
Add & Manage Slides in Presentations via C# API
Aspose.Slides for .NET has included complete support for handling slides in PowerPoint presentations inside .NET applications. The library has included numerous features for working with slides such as adding new slides to a presentation, accessing an existing slide, removing unwanted slides, cloning slides, copying existing slides, comparing two slides, managing slide layout, slide transition, converting slides to Bitmap, converting slides to images with custom sizes and many more. Additionally, you can also convert slides with notes and comments to images or export all slides to images with just a couple of lines of code.
Clone a Slide via C# API
// Instantiate Presentation class that represents a presentation file
using (Presentation pres = new Presentation("CloneWithinSamePresentationToEnd.pptx"))
{
// Clone the desired slide to the end of the collection of slides in the same presentation
ISlideCollection slds = pres.Slides;
slds.AddClone(pres.Slides[0]);
// Write the modified presentation to disk
pres.Save("Aspose_CloneWithinSamePresentationToEnd_out.pptx", SaveFormat.Pptx);
}
Add Animations to PowerPoint Presentation via C#
Aspose.Slides for .NET allow software developers to apply different types of animation to their presentations with ease. PowerPoint animation plays an important role in order to make presentations eye-catching and attractive for audiences. The library supports 150+ animation effects that can be easily applied to shapes, charts, tables, OLE Objects, and other presentation elements. Additionally, it is also to combine several behaviors to create your own custom animations.
Add Animation Effect to a Single Paragraph via .NET API
using (Presentation presentation = new Presentation(dataDir + "Presentation1.pptx"))
{
// select paragraph to add effect
IAutoShape autoShape = (IAutoShape)presentation.Slides[0].Shapes[0];
IParagraph paragraph = autoShape.TextFrame.Paragraphs[0];
// add Fly animation effect to selected paragraph
IEffect effect = presentation.Slides[0].Timeline.MainSequence.AddEffect(paragraph, EffectType.Fly, EffectSubtype.Left, EffectTriggerType.OnClick);
presentation.Save(dataDir + "AnimationEffectinParagraph.pptx", SaveFormat.Pptx);
}